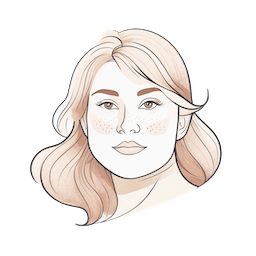
Fundamentals
Workflows in Flawless are just regular Rust functions annotated with the
#[workflow("<name>")]
macro.
#[workflow("test")]
fn workflow() {
info!("Hello from workflow!");
}
Input arguments
Workflows can also take one input argument.
use flawless::{log::info, workflow};
#[workflow("test")]
fn workflow(name: String) {
info!("Hello {name}!");
}
Running it with:
$ cargo flw run test --input '"Flawless"'
will print out something like:
[2023-12-14T16:30:53Z INFO] [ID] Hello Flawless!
The input can be any structure that implements the serde::Deserialize
trait. And it's
passed to the workflow as a JSON value. In this example the input is a JSON string
("Flawless"
).
Exposing data from a workflow to the outside world
The best way to expose some data to the outside world is by using the
set_data(value)
function. The value can be any structure that implements the serde::Serialize
trait and
is going to be accessible as a JSON value.
The structure of the value can change during the execution.
use flawless::{exe::set_data, workflow};
#[workflow("add")]
fn workflow(input: Input) {
set_data(&(input.a + input.b));
}
Run it with:
$ cargo flw run add --input '{"a": 7, "b": 6}'
You can check out the output using the data
command or through the
HTTP API.
$ flawless workflow data <ID>
13
Shared global state
Workflows can define and share state globally using the
State
API.
The following example shows a simple workflow that increments a global number:
use flawless::{state::State, workflow};
use serde::{Deserialize, Serialize};
#[derive(Serialize, Deserialize, Default, Debug)]
struct AppState {
num: i32,
}
#[workflow("inc")]
fn inc() {
let mut state: State<AppState> = State::new("app_state");
state.write(|s| {
s.num += 1;
});
}
Each global state is identified by a unique name. The access to the state is exclusive, if two workflows are trying to access is, one of them will be blocked until the other is done with it.
Working with secrets
Secrets are variables that you can set with:
$ flawless secret set <VARIABLE> <VALUE>
Inside the workflows, you can retrieve a secret using the secret::get()
function.
let secret = flawless::secret::get("VARIABLE");